Overview
TAble is your handy desktop app, optimized for TAs who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI)! I have added the functionality for TAs to arrange consults and view all upcoming events in a calendar! The GUI of TAble was created with JavaFX and written in Java, and I have written about 4 kLoC.
Summary of contributions
-
Major enhancement: Enabled support for Consults
-
What it does: The user is able to add/edit/delete/list all
Consults
which he has scheduled with a currentStudent
. -
Highlights: A separate consult panel that displays all of the user’s
Consults
exist for the user to easily keep track of all his consults. Any consults that are overdue are also highlighted in red, to remind the user to delete these outdated consults.
-
-
Major enhancement: Added a calendar view for TAble.
-
What it does: Added a calendar view that displays all the
Consults
,Tutorials
andReminders
for the user. -
Highlights: This calendar is able to show the number of
Consults
,Tutorials
andReminders
that occurs on a given day in one view, without any information being ommitted. -
Credits: {Code for the Calendar Window adapted from https://github.com/SirGoose3432/javafx-calendar }
-
-
All code contributed: [RepoSense - Code Report]
-
Other contributions:
-
Project management:
-
Raised issues that needed to be solved and provided some solutions to these problems. [Issues raised/discussed]
-
-
Documentation:
-
Contributed in the
Consult
andCalendar
sections of the User Guide: -
Contributed in the
Consult
andCalendar
sections of the Developer Guide: which includes the class diagram forConsults
, activity diagram foraddConsult
command and a sequence diagram for the creation of the Calendar. More details on these are covered below.
-
-
Tools:
-
Helped to set up Travis-CI and Netifly.
-
-
Summary of contributions:
-
Created over [35 pull requests] on GitHub
-
Reviewed over [36 pull requests]on GitHub
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Consults Feature (Written by Han Ching)
The consults that are overdue will appear in red to highlight that these consults are ready to be deleted! |
Adding a consult: addConsult
Add a consultation slot at the given time, date and place.
Format: addConsult student/STUDENT_INDEX beginDateTime/BEGIN_DATE_TIME endDateTime/END_DATE_TIME place/PLACE
Consults will be automatically sorted according to their date and time, with the earliest at the top of the list. |
Example:
-
addConsult student/1 beginDateTime/2020-03-03 10:00 endDateTime/2020-03-03 12:00 place/Deck
Editing a consult: editConsult
Edit the time, date or place of an existing consultation slot.
Format: editConsult INDEX [beginDateTime/BEGINDATETIME] [endDateTime/ENDDATETIME] [place/PLACE]
Example:
-
editConsult 1 beginDateTime/2020-03-03 15:00
Edits the beginning time of the 1st consult to be at 2020-03-03, 15:00 hours i.e 3.00 p.m. -
editConsult 2 place/SR3
Edits the place of the 2nd consult to be at SR3.
Listing all consults : listConsult
Shows a list of all consultations in TAble.
Format: listConsult
Deleting a consult: deleteConsult
Removes an existing consultation slot.
Format: deleteConsult INDEX
Example:
-
listConsult
deleteConsult 2
Deletes the 2nd consultation in TAble.
Clearing all consults : clearConsults
Clears all consultations slots in TAble.
Format: clearConsults
Locating consultations by date or place: findConsult
[coming in v2.0]
Finds consultations whose date or place match any of the given keywords.
Format: findConsult [DATE] [PLACE]
Examples:
-
findConsult 03-03-2020
Returns all consults on 03-03-2020 -
findConsult SR3
Returns any consults that are held at SR3
Calendar Feature (Written by Han Ching)
Display the calendar window: viewCalendar
Brings up the calendar window.
Format: viewCalendar
Close the calendar window: closeCalendar
Closes the calendar window.
Format: closeCalendar
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Consult feature (Written by Han Ching)
TAble allows NUS SoC teaching assistants to track and record all their consultations scheduled with their students.
Implementation
A Consult
class stores all of the relevant information of consults. The class diagram below shows how all the different components interact to allow the consult feature to function. Note that the XYZConsultCommand and XYZConsultCommandParser refers to all Consult related commands like add, edit, delete etc.
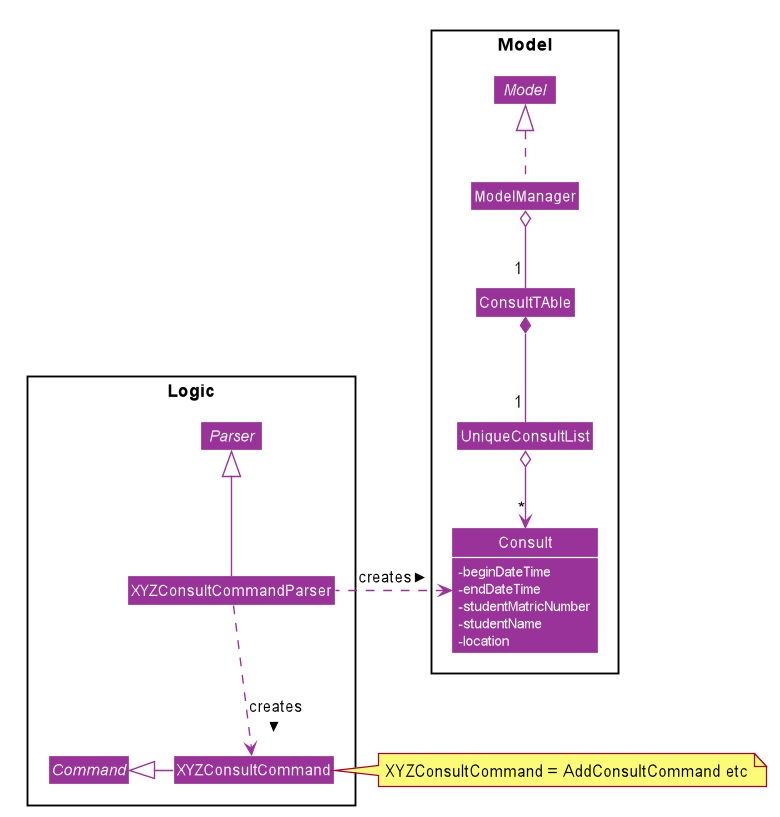
A consultation slot is represented by the Consult
class which contains 5 key attributes, the beginDateTime
, endDateTime
, location
, studentMatricNumber
and studentName
.
The beginDateTime
and endDateTime
attributes are represented using Java’s LocalDateTime
class. The studentMatricNumber
and studentName
attributes are classes that belong to a certain Student
. The studentMatricNumber
is used to uniquely identify the Student
involved in the consultation, while the studentName is used in the front end of the application, displayed to the TA.
The XYZConsultCommand
class represent classes that extend the abstract class Command
and allows the TA to add, edit, delete, view and clear consultations added to TAble. These XYZConsultCommandS
are created by the respective XYZConsultCommandParserS
.
A ConsultTAble
that has a UniqueConsultList
stores and manages all ConsultS
. When a Consult
is added to the ConsultTAble, there will first be a check that the Consult
does not already exist in the UniqueConsultList
.
Given the class diagram and the understanding of how the Consult
class interacts with other classes, let us examine how an addConsult
command behaves, by using the following activity diagram of the system.
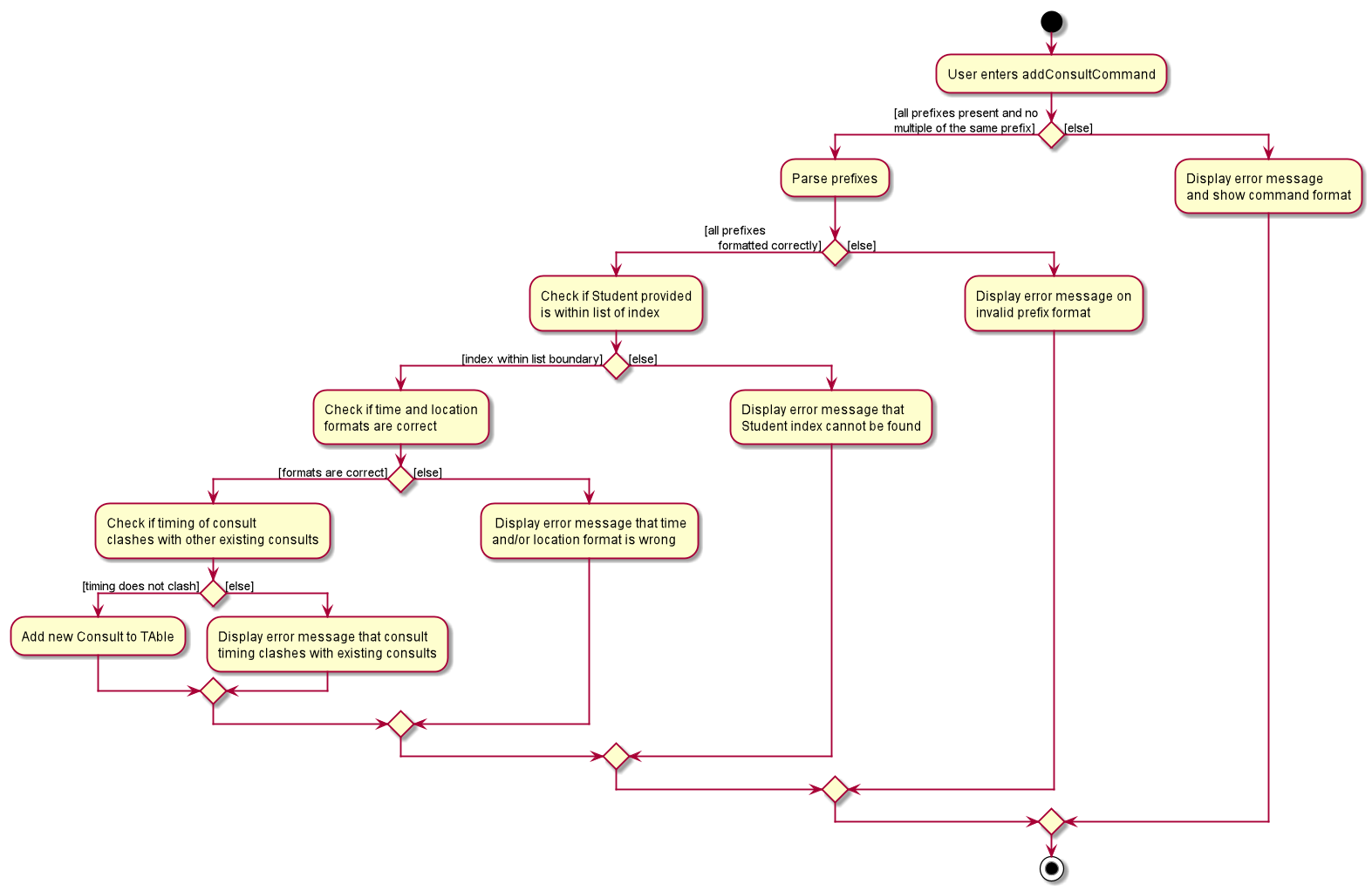
Consult
featureThe workflow above shows how a Consult
is added and the various checks that occurs at the different points throughout the workflow.
To summarize the above activity diagram, there are 5 key checks which TAble checks for when the user is adding a consult
. Firstly, TAble checks if all 4 prefixes are present in the command.
Then, TAble checks if all prefixes present are formatted correctly. Following which, TAble will check if each of the data passed for the prefixes are in the right format. Firstly, it checks if
the Student
index provided is within the range of the Student
list. Then, it checks if the timing and Location
provided are in the correct format. Lastly, and most importantly, it checks if the
Consult
timing provided clashes with other existing Consult
timings.
Design Considerations
Consult storing Matric Number and Name
Alternative 1 (Current Choice): Make the consult store the matric number and name of the student that is attending the consult. |
Alternative 2 : Make the consult store the entire Student class that is attending the consult. |
|
Pros |
It is easier to implement and coupling will be reduced as |
It is easier to visualise as the entire |
Cons |
It is not as intuitive as currently, the |
It will be harder to test due to the high degree of coupling. |
Reason for choosing Alternative 1: Due to the time constraint of this project, our group has decided to choose alternative 1, as it not only reduces coupling, but is sufficient for us to uniquely identify the Student
participating in the consult
as the MatricNumber
would be unique.
Sorting the consults
Alternative 1 (Current Choice): Automatically sorts the consult list by the time of the consult, whenever a consult is added |
Alternative 2 : Sorts the consult list only when user enters a sort command |
|
Pros |
It is intuitive for the consult list to be sorted according to their date and time. |
User can decide how they want the list to be sorted (e.g. by alphabetical order for location). |
Cons |
User are not able to sort the list according to their preferences. |
User have to type the sort command each time a new consult is added. |
Reason for choosing Alternative 1: We believe that automatically sorting the consult list provides a better user experience as the user can immediately see the upcoming consults in the panel, without having to type a sort command.
Calendar feature (Written by Han Ching)
TAble allows NUS SoC teaching assistants to bring up a calendar view of all their upcoming consults, tutorials and reminders.
Implementation
The key command which users can call is the viewCalendar
command.
Most functions to display the calendar window are called within the CalendarWindow
class, as it is the main class that drives the calendar view.
The CalendarWindow
class extends UiPart<Stage>
, and represents the calendar using a GridPane
as the skeleton of the calendar, with individual dates being populated by a CalendarDay
class which extends UiPart<Region>
.
The CalendarDay
has a StackPane
that displays the number of ConsultS
, TutorialS
and ReminderS
each day.
The following sequence diagram demonstrates how the Calendar is initialised, each time the TAble app is set up.
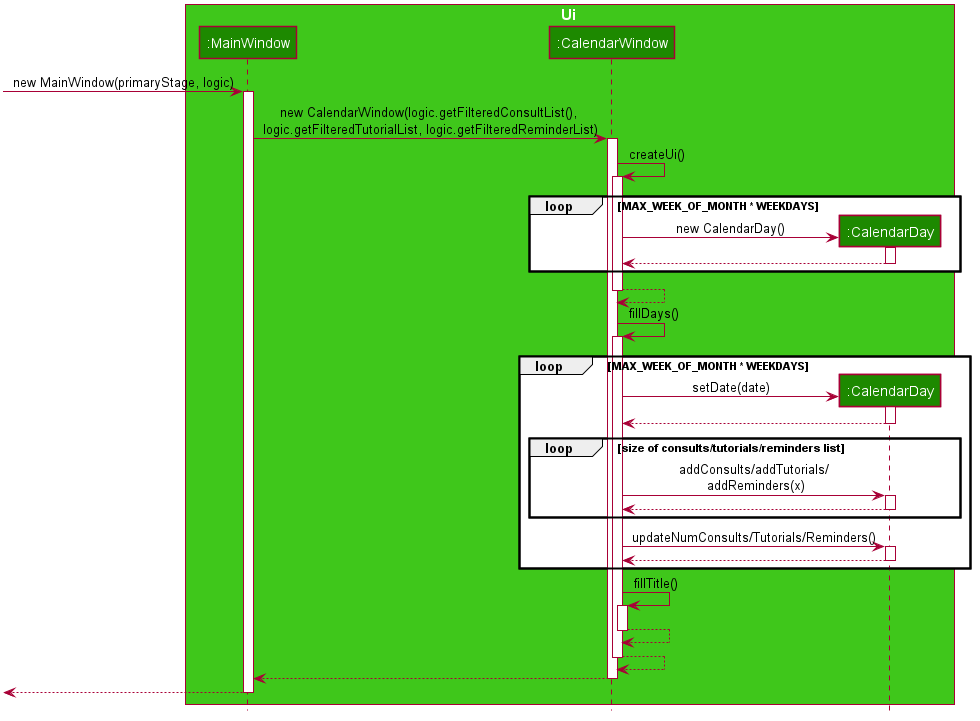
The above sequence diagram illustrates how the Calendar is initialised when the TAble app is start up. Thus, whenever the user inputs the viewCalendar
command,
this calendar window is brought up, similar to how the Help window is brought up as well. The calendar window brought up will show the calendar view of the current month and year.
The calendar window also stores the UniqueConsultList
, UniqueTutorialList
and the UniqueReminderList
. As seen in the sequence diagram above, the calendar window checks for the consults, tutorials and reminders in the 3 lists, to see if they are on a particular date.
If they occur on that date, the StackPane
in CalendarDay
will be updated to reflect the corresponding number of consults, tutorials and reminders.
As seen in the screenshot below, the number of reminders, tutorials and consults will not clash, as they take up the top, middle and bottom rows respectively.
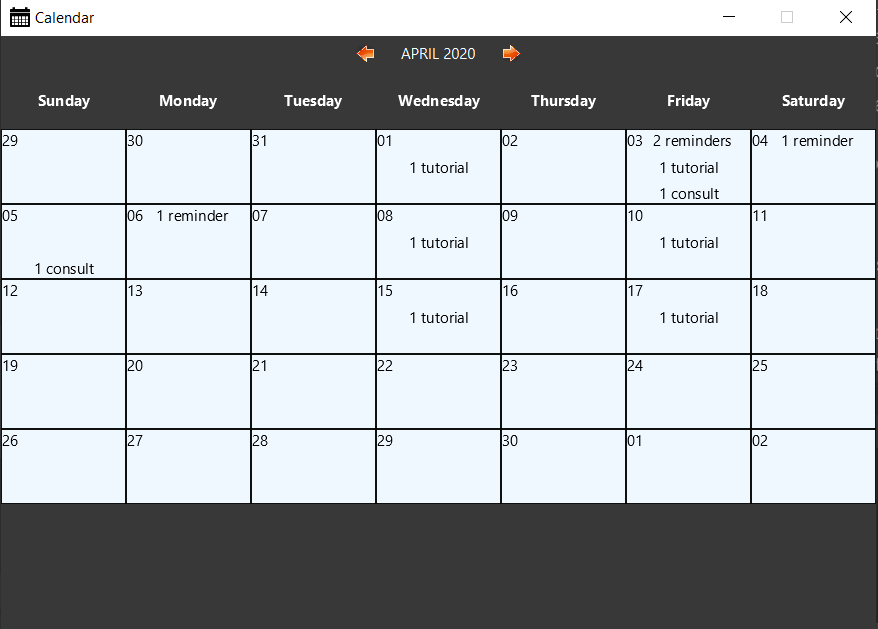
Design Considerations
Displaying calendar as a separate window
Alternative 1 (Current Choice): Displaying the calendar view as a separate window. |
Alternative 2 : Having a calendar view permanently by the side of TAble. |
|
Pros |
It is clearer and less visually clunky for the user, as information overload is prevented. |
It is more intuitive, as the user can always view the calendar at the side for his upcoming schedules. |
Cons |
Lower user experience as the user has to type in a command to view the calendar. |
The UI may be too cluttered as there are already alot of information being displayed currently. |
Reason for choosing Alternative 1: We believe that our current implementation of the UI has all of TAble’s core features, and the calendar view can and should be implemented as a separate window to prevent visual clutter. The command to view the calendar window command is also short and intuitive to remember, thus the user experience would likely not be significantly affected.
Use Cases
Use case: Edit consult (U09)
MSS
-
User requests to update either the time, date or place of an existing consult
-
System updates the existing consult according to the User’s request
Use case ends.
Extensions
-
1a. The consult does not exist.
-
1a1. System shows an error message that the consult does not exist.
Use case ends.
-
-
1b. The time, date or place provided is in the wrong format.
-
1b1. System shows an error message that time, date or place must be provided in the correct format.
Use case ends.
-
Use case: Delete consult (U10)
MSS
-
User requests to delete an existing consult
-
System removes the existing consult from the database
Use case ends.
Extensions
-
1a. The consult does not exist.
-
1a1. System shows an error message that the consult does not exist.
Use case ends.
-
-
1b. The user enters the delete consult command in the wrong format.
-
1b1. System shows an error message that the wrong delete consult command format was given.
Use case ends.
-