Overview
TAble is your handy desktop app, optimized for TAs who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI)! Mark attendance, arrange consults, add module notes, and more with TAble! It has a GUI created with JavaFX and written in Java, and has about 20 kLoC.
Summary of contributions
-
Major enhancement: added the ability to insert Module description/links/time
-
What it does: Represents module information critical to a Teaching Assistant’s role in their work, from notes of the module to handling module links.
-
Justification: Teaching Assistants need to view general module information and associate Tutorials with modules. This helps consolidate general information about the module (through its notes and module links).
-
Highlights: Module links can be stored in TAble and shared easily with the command to copy links into the user’s clipboard. Additionally, note of the module can also be updated to store important information like grade breakdown.
-
-
Minor enhancement: Add command to export tutorial attendance to CSV file
-
What it does: Tutorial attendance of students as stored in TAble will be exported into a CSV file to a path defined by the user, showcasing the students' names, matriculation number, emails and attendance for the weeks of the tutorial.
-
Justification: After TAs mark attendance for their students in TAble, TAs will usually need to update the attendance into another document. This command allows TAs to easily copy the data over into another spreadsheet where necessary.
-
Highlights: The generated CSV file should follow the same format as what is given when exporting student data on LumiNUS.
-
-
Code contributed: As seen on RepoSense
-
Other contributions:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Module Feature (Written by Nigel Neo)
Add Module into database: addMod
Adds a module into TAble.
Due to naming restrictions in java, all code references to modules are named Mod instead of Module. |
Format: addMod modCode/MODULE_CODE modName/MODULE_NAME
Example:
-
addMod modCode/CS1010S modName/Programming Methodology
Delete Module from database: deleteMod
Deletes a module from TAble.
Format: deleteMod modCode/MODULE_CODE
Example:
-
addModule modCode/CS1010S modName/Programming Methodology
Edit Module note: noteMod
Edits corresponding note of module.
Format: noteMod modCode/MODULE_CODE modNote/NOTE
Example:
-
noteModule modCode/CS1010S modNote/How cool is that?
When viewing the module info for CS1010S, the note will be updated to show "How cool is that?".
Add link to module with appropriate name: addModLink
Edit module to include links to appropriate websites (e.g. Google Drive Folder, Module Website etc.)
Format: addModLink modCode/MODULE_CODE modLink/LINK modLinkName/NAME
Example:
-
addModLink modCode/CS1231 modLink/https://comp.nus.edu.sg/~cs1231 modLinkName/Module Website
When viewing the module page for CS1231, there will be an additional label with "Module Website" and points to https://comp.nus.edu.sg/~cs1231.
Clear all module links from module in database: clearModLink
Removes all module links from a module in TAble.
Format: clearModLink modCode/MODULE_CODE
Example:
-
clearModLink modCode/CS1231
When viewing the module page for CS1231, there will not be any module links shown.
Copy Module Link into Clipboard: copyModLink
Copies a module link from the links currently associated with a module. Index corresponding to module link can be seen when viewing the module info using viewModInfo
.
Format: copyModLink INDEX modCode/MODULE_CODE
Example:
-
copyModLink 1 modCode/CS1231
The first module link for CS1231 is copied into the user’s clipboard.
View Module: viewModInfo
Opens the view for the selected module to view module description, links and (coming in v2.0) lecture timeslots.
Format: viewModInfo modCode/MODULE_CODE
Add lecture timeslot: addModLecture
[coming in v2.0]
Adds lecture timeslot to module. This is to be integrated with the future calendar display feature.
Format: addModLecture modCode/MODULE_CODE d/DAY from/TIME to/TIME p/LOCATION
Example:
-
addModLecture modCode/CS1010S d/WED from/1400 to/1600 p/LT27
Clear all lecture timeslots: clearModLecture
[coming in v2.0]
Clears all lectures associated with a certain module.
Format: clearModLecture modCode/MODULE_CODE
Example:
-
clearModLecture modCode/CS1010S
Tutorial Feature
Export tutorial attendance to csv file: exportAttendance
(Written by Nigel Neo)
Exports the attendance data of all enrolled students in a tutorial group to a csv file, where the filepath is specified by the user.
Format: exportAttendance tutorialIndex/INDEX csvFilePath/FILEPATH
Example:
-
exportAttendance tutorialIndex/1 csvFilePath/./data/attendance.csv
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Module feature (Written by Nigel Neo)
TAble allows NUS SoC teaching assistants to track and record all modules that they are teaching. Users can leave notes on the modules that they are teaching and store a list of links for the module that are useful for reference.
Implementation
A module can be stored as a Mod object in TAble (renamed due to naming restrictions on Java keywords). The main components are the module code, which is used to identify unique modules, and module name. Mod can also store the user’s notes of the module through its description parameter, as well as a list of module links which are relevant to the course.
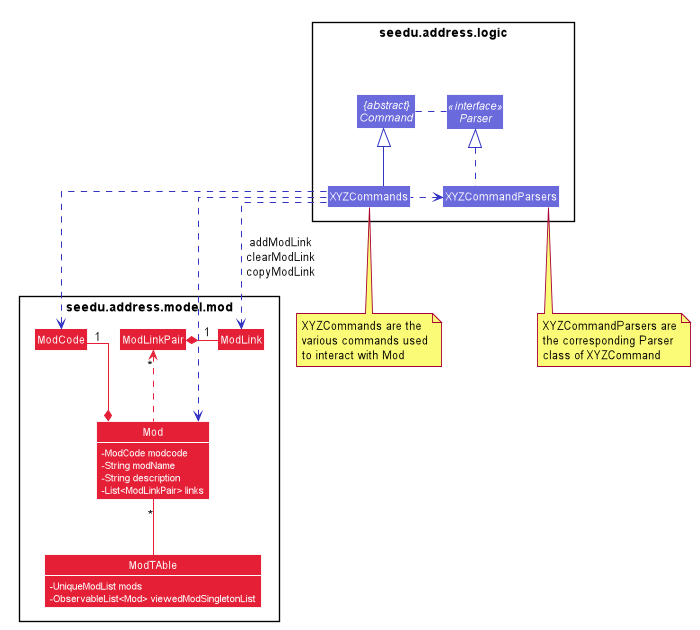
The following sequence diagram shows how notes of a module can be updated with the noteMod
command. Each command is parsed into a ModCode object and the note stored as string. The ModCode is then used to retrieve the appropriate Mod object in model
. Next, this is used in the Mod constructor to come up with a new Mod with the given note. This new Mod replaces the original Mod in model and the command returns a string indicating success.
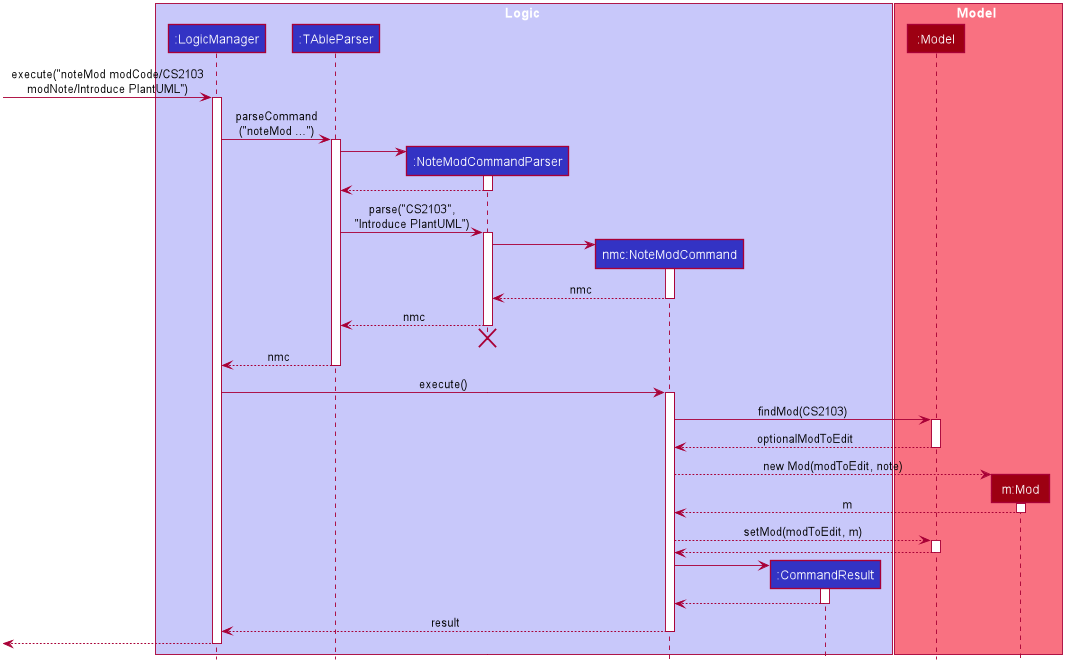
Module links are stored as ModLink objects in TAble. The collection of ModLinks are stored as List<ModLinkPair>
(where a ModLinkPair is actually a class wrapper for a Pair<String, ModLink> object) since each module link can be described differently. This allows for more flexibility in naming the module links, rather than solely using an index based notation, and ensures that the order of addition into the collection is maintained. The activity diagram of adding module links is shown below.
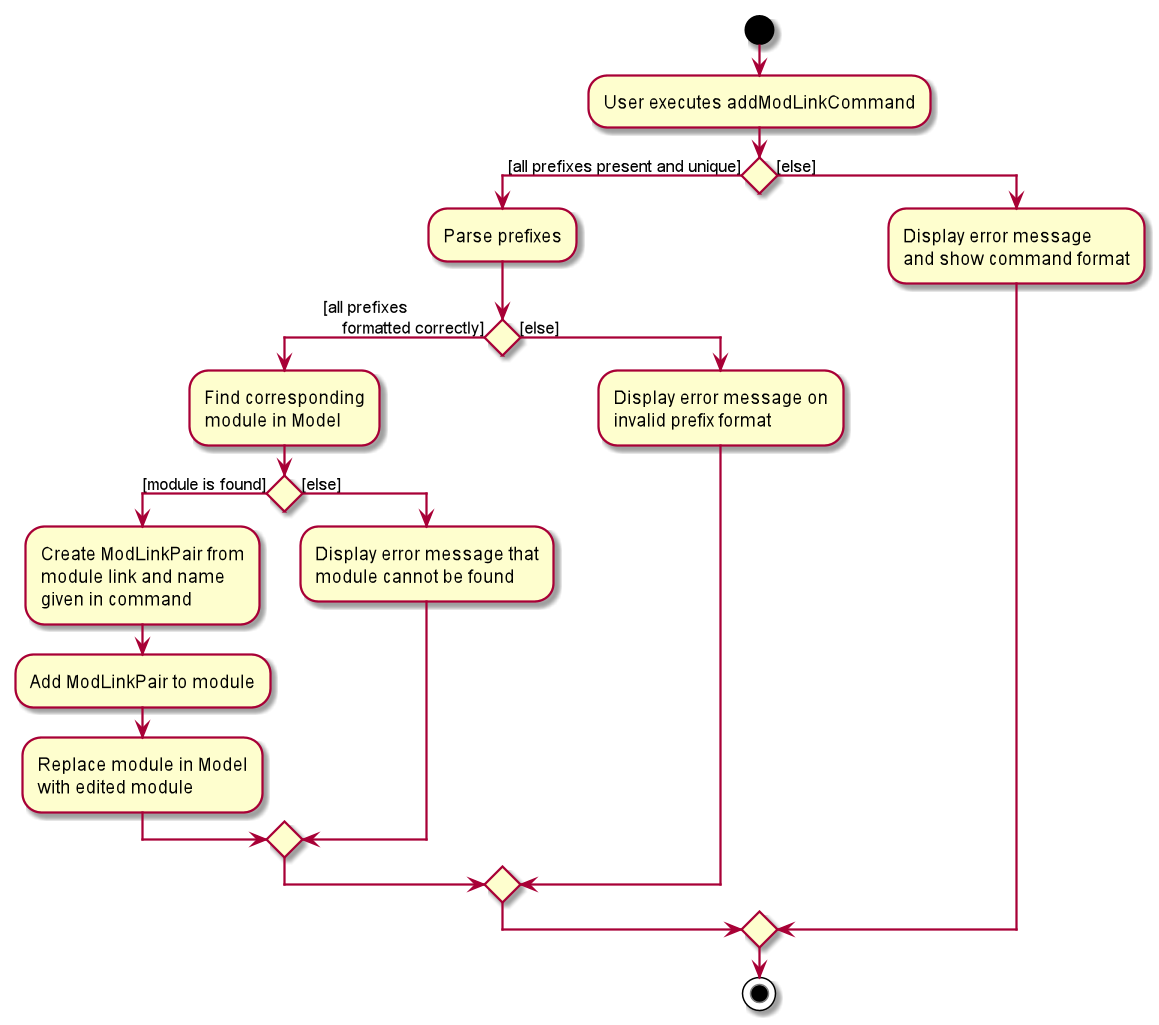
Users can then view the information associated with a Mod object by using the viewModInfo
command, and copy module links with the copyModLink
command. Discussion on the copyModLink command is shown below.
Design Considerations
Unable to delete module with associated tutorials
Alternative 1: (Current Choice) Disable deletion of module with associated tutorials |
Alternative 2 : Delete module together with its associated tutorials |
|
Pros |
Informs user if there are any dependencies between module to be deleted and tutorials in TAble. |
Fast deletion of module and related objects. |
Cons |
User needs to individually delete associated tutorials before module can be deleted. |
Information in TAble will be lost easily as user may not be aware of such an association. As there is no undo command, it will not be easy to undo this command and revert TAble to its original state. |
Reason for choosing Alternative 1: On a user design perspective, it is not advisable to delete all objects associated with module, as the user may be unaware of these associated objects. By individually confirming the objects to be deleted, the user is made more aware of any information that they may want to export or save first before deleting said information.
ModLink behaviour
Alternative 1: (Current Choice) Copy link into user’s clipboard |
Alternative 2 : Open a new browser page |
|
Pros |
Allows for easier access and sharing of link. |
Fast access to module’s weblink by directly opening a browser. |
Cons |
User needs to open a browser before accessing link. |
Very distracting when opening link, as focus changes from TAble to browser unexpectedly (depending on when browser loads). |
Reason for choosing Alternative 1: On a user design perspective, it is less jarring to copy link into the user’s clipboard, since the user can choose when they want to access the link and have the flexibility to share the link to others. Additionally, opening a new browser page requires more complicated code that depends on another program.